Introduction to PHP
PHP is an acronym for “PHP: Hypertext Preprocessor”, and is widely-used, open source scripting language.
-
- PHP 8.2 is the latest version
- PHP was created by Rasmus Lerdorf in 1994
This language is powerful tool for making dynamic and interactive Web pages. PHP code is executed on the server.
-
- It is an integrated with the number of popular databases, including MySQL, PostgreSQL, Oracle, sybase, etc
Why PHP?
-
- PHP runs on various platforms (Windows, Linux, Unix, Mac OS X, etc.)
- PHP is compatible with almost all servers used today (Apache, IIS, etc.)
- PHP supports a wide range of databases
- PHP is free. Download it from the official PHP resource: www.php.net
Popular Frameworks & CMS
-
- Joomla
- Magento2
- WordPress
- CakePHP
- Laravel
- CodeIgnitor
Pre-requisites for Learning PHP
The following are the pre-requisites before starting learning PHP:-
-
- HTML
- CSS (Basic)
- Javascript (Optional)
Install PHP
To install PHP, we need following available.
-
- Install Server
- Install PHP
- Install MySQL
We will suggest you to install AMP (Apache, MySQL, PHP) software stack. It is available for all operating systems. There are many AMP options available in the market that are given below:
-
- WAMP for Windows
- LAMP for Linux
- MAMP for Mac
- SAMP for Solaris
- FAMP for FreeBSD
- XAMPP (Cross, Apache, MySQL, PHP, Perl) for Cross Platform: It includes some other components too such as FileZilla, OpenSSL, Webalizer, Mercury Mail, etc.
What is a PHP File?
-
- PHP files can contain text, HTML, CSS, JavaScript, and PHP code
- PHP code is executed on the server, and the result is returned to the browser as plain HTML
- PHP files have extension “
.php
“
Basic PHP Syntax
A PHP script can be placed anywhere in the document. A PHP script starts with <?php
and ends with ?>
:
<?php
// PHP code goes here
?>
The default file extension for PHP files is “.php
“.
A PHP file normally contains HTML tags, and some PHP scripting code.
Below, we have an example of a simple PHP file, with a PHP script that uses a built-in PHP function “echo
” to output the text “Hello World!” on a web page:
Example
<!DOCTYPE html> <html> <body> <h1>My first PHP page</h1> <?php echo "Hello World!"; ?> </body> </html>
;
).PHP Comments
PHP Comments is one of the most important parts of the code. A comment is simply text that is ignored by the PHP engine. PHP comments don’t affect the code.
Purpose of Comments
There are the following uses of comments:-
-
- By using comments, the developer can easily understand the code.
- These comments are simply a description of the PHP script or code.
- To make the code more readable.
- It may help other developer (or you in the future when you edit the source code) to understand what you were trying to do with the PHP.
In HTML, we see there is only one type of comment. While PHP has two types of comments i.e.
-
- Single Line Comment
- Multiple Line Comment
1. Single Line Comment
The Single-line comments are used to tell the developer to ignore everything which comes to the right side of that syntax of single-line comment.
We can use single-line comments by the following two ways:
-
//
#
There is no need to end the single-line comment.
Example
<?php
// This is a single line comment
# This is also a single line comment
echo "Hello, world!";
?>
2. Multiple Line Comment
The Multiple PHP comment can be used to comment out large blocks of code(we can even comment paragraphs in PHP script). The multiple line PHP comment begins with /*
and ends with */
.We must end the multiple line comment.
However to write multi-line comments, start the comment with a slash followed by an asterisk (/*
) and end the comment with an asterisk followed by a slash (*/
), like this:
Example
<?php
/*
This is a multiple line comment block
that spans across more than one line
*/
echo "Hello, world!";
?>
PHP Variables
Variables are used to store data, like string of text, numbers, etc. Variable values can change over the course of a script. Here’re some important things to know about variables:
-
- In PHP, a variable does not need to be declared before adding a value to it.
- PHP automatically converts the variable to the correct data type, depending on its value.
- After declaring a variable it can be reused throughout the code.
- The assignment operator (
=
) used to assign value to a variable.
In PHP, variable can be declared as: $var_name = value;
Example
<?php
// Declaring variables
$txt = "Hello World!";
$number = 10;
// Displaying variables value
echo $txt; // Output: Hello World!
echo $number; // Output: 10
?>
In the above example we have created two variables where first one has string assigned with a value and the second has assigned with a number.
Naming Conventions for PHP Variables
These are the following rules for naming a PHP variable:
-
- All variables in PHP start with a
$
sign, followed by the name of the variable. - A variable name must start with a letter or the underscore character
_
. - A variable name cannot start with a number.
- A variable name in PHP can only contain alpha-numeric characters and underscores (
A-z
,0-9
, and_
). - A variable name cannot contain spaces.
- All variables in PHP start with a
PHP Case Sensitivity
In PHP, keywords (e.g. if
, else
, while
, echo
, etc.), classes, functions, and user-defined functions are not case-sensitive.
In the example below, all three echo statements below are equal and legal:
<!DOCTYPE html> <html> <body> <?php ECHO "Hello World!<br>"; echo "Hello World!<br>"; EcHo "Hello World!<br>"; ?> </body> </html>
Note: However; all variable names are case-sensitive!
Look at the example below; only the first statement will display the value of the $color
variable! This is because $color
, $COLOR
, and $coLOR
are treated as three different variables:
<!DOCTYPE html> <html> <body> <?php $color = "red"; echo "My car is " . $color . "<br>"; echo "My house is " . $COLOR . "<br>"; echo "My boat is " . $coLOR . "<br>"; ?> </body> </html>
However the keywords, function and classes names are case-insensitive. As a result calling the gettype()
or GETTYPE()
produce the same result.
Example
<?php
// Assign value to variable
$color = "blue";
// Get the type of a variable
echo gettype($color) . "<br>";
echo GETTYPE($color) . "<br>";
?>
If you try to run the above example code both the functions gettype()
and GETTYPE()
gives the same output, which is: string.
PHP Data Types
Variables can store data of different types, and different data types can do different things.
PHP supports the following data types:
-
- String
- Integer
- Float (floating point numbers – also called double)
- Boolean
- Array
- Object
- NULL
- Resource
PHP String
A string is a sequence of characters, like “Hello world!”. A string can be any text inside quotes. You can use single or double quotes:
Example
<?php $x = "Hello world!"; $y = 'Hello world!';echo $x; echo "<br>"; echo $y; echo gettype($x); ?>
PHP Integer
An integer data type is a non-decimal number between -2,147,483,648 and 2,147,483,647.
Rules for integers:
-
- An integer must have at least one digit
- An integer must not have a decimal point
- An integer can be either positive or negative
- Integers can be specified in: decimal (base 10), hexadecimal (base 16), octal (base 8), or binary (base 2) notation
In the following example $x is an integer. The PHP var_dump() function returns the data type and value:
Example
<?php
$x = 5985;
echo gettype($x);
?>
PHP Float
A float (floating point number) is a number with a decimal point or a number in exponential form.
In the following example $x is a float. The PHP var_dump() function returns the data type and value:
Example
<?php
$x = 10.365;
echo gettype($x);
?>
PHP Boolean
A Boolean represents two possible states: TRUE or FALSE.
$x = true;
$y = false;
Booleans are often used in conditional testing.
PHP Array
An array stores multiple values in one single variable.
In the following example $cars is an array. The PHP var_dump() function returns the data type and value:
Example
<?php
$cars = array("Volvo","BMW","Toyota");
var_dump($cars);
?>
PHP Object
Classes and objects are the two main aspects of object-oriented programming.
A class is a template for objects, and an object is an instance of a class.
When the individual objects are created, they inherit all the properties and behaviors from the class, but each object will have different values for the properties.
Let’s assume we have a class named Car. A Car can have properties like model, color, etc. We can define variables like $model, $color, and so on, to hold the values of these properties.
When the individual objects (Volvo, BMW, Toyota, etc.) are created, they inherit all the properties and behaviors from the class, but each object will have different values for the properties.
If you create a __construct() function, PHP will automatically call this function when you create an object from a class.
Example
<?php class Employee { public $name; public $designation; public function __construct($name, $designation) { $this->name= $name; $this->designation= $designation; } public function message() { return "Employee is a " . $this->name. " " . $this->designation. "!"; } } $employee = new Employee ("Dev", "SSE"); echo $employee->message(); echo "<br>"; $employee = new Employee ("Tinku", "TL"); echo $employee -> message(); ?>
PHP NULL Value
Null is a special data type which can have only one value: NULL. A variable of data type NULL is a variable that has no value assigned to it.
Tip: If a variable is created without a value, it is automatically assigned a value of NULL.
Variables can also be emptied by setting the value to NULL:
Example
<?php
$x = "Hello world!";
$x = null;
echo gettype($x);
?>
PHP Resource
The special resource type is not an actual data type. It is the storing of a reference to functions and resources external to PHP. A common example of using the resource data type is a database call.
Concatenate String, Variable
Lets learn how to concatenate String, Variable in PHP. The concatenate term in PHP refers to joining multiple strings into one string; it also joins variables.
In PHP, concatenation is done by using the concatenation operator (".")
which is a dot. The php developers widely use the concatenation operator, it helps them to join string in their day to the day programming task.
-
- PHP Concatenate Operator
- Concatenate Two Strings in PHP
- Concatenate Two Variables in PHP
- Concatenating variable with string in PHP
1. PHP Concatenate Operator
PHP offers two types of string operators to deal with string concatenation.
Operator | Detail |
---|---|
(".") |
The dot concatenation operator in PHP concatenate its right and left arguments. |
('.=') |
This second concatenation operator helps in appending the right side argument to the left side argument. |
2. Concatenate Two Strings in PHP
You can combine two strings in PHP using the concatenate operator, check out the code example below:
<?php
$string1 = 'Sonal';
$string2 = 'from TheCoachSMB';
$name_str = $string1 . ' ' . $string2;
echo $name_str;
?>
// Result: Sonal from TheCoachSMB
3. Concatenate Two Variables in PHP
n this php tutorial, we will now learn to concatenate two variables, in the following example we have declared the two variables $var_1
and $var_2
. Then in the $var_3
variable we are adding the content of both the variables:
<?php
$var_1 = "ABC";
$var_2 = "XYZ";
$var_3= $var_1." ".$var_2." ";
echo $var_3;
?>
Output will be:
ABC XYZ
4. Concatenating variable with string in PHP
In this example we are Concatenating single variable with string:
Since PHP 8.0, defining case-insensitive constants has been removed. The third parameter of the define
function is ignored and using case-insensitive constant provides a fatal error.
Constant vs Variables
Constant | Variables |
---|---|
Once the constant is defined, it can never be redefined. | A variable can be undefined as well as redefined easily. |
A constant can only be defined using define() function. It cannot be defined by any simple assignment. | A variable can be defined by simple assignment (=) operator. |
There is no need to use the dollar ($) sign before constant during the assignment. | To declare a variable, always use the dollar ($) sign before the variable. |
Constants do not follow any variable scoping rules, and they can be defined and accessed anywhere. | Variables can be declared anywhere in the program, but they follow variable scoping rules. |
Constants are the variables whose values can’t be changed throughout the program. | The value of the variable can be changed. |
By default, constants are global. | Variables can be local, global, or static. |
PHP Operators
Operator is a symbol that tell the compiler which operations (arithmetic, comparision, etc) to be performed between the operands.
Example:-
$num=10+20;//+ is the operator and 10,20 are operands
In the above example, + is the binary + operator, 10 and 20 are operands and $num is variable.
PHP divides the operators in the following groups:
-
- Arithmetic operators
- Assignment operators
- Comparison operators
- Increment/Decrement operators
- Logical operators
- String operators
- Array operators
- Conditional assignment operators
1. PHP Arithmetic Operators
The PHP arithmetic operators are used with numeric values to perform common arithmetical operations, such as addition, subtraction, multiplication etc.
Operator | Name | Example | Result |
---|---|---|---|
+ | Addition | $x + $y | Sum of $x and $y |
– | Subtraction | $x – $y | Difference of $x and $y |
* | Multiplication | $x * $y | Product of $x and $y |
/ | Division | $x / $y | Quotient of $x and $y |
% | Modulus | $x % $y | Remainder of $x divided by $y |
** | Exponentiation | $x ** $y | Result of raising $x to the $y’th power |
Example:
<?php echo "a = ". $a = 20; echo "<br>b = ". $b = 5; echo "<h2>Arithmetic Oprations</h2>"; echo "<h3>Addtion</h3>"; echo $result = $a + $b; echo "<h3>Subtraction</h3>"; echo $result = $a - $b; echo "<h3>Multiplication</h3>"; echo $result = $a * $b; echo "<h3>Division</h3>"; echo $result = $a / $b; echo "<h3>Modulus</h3>"; echo $result = $a % $b; echo "<h3>Exponentiation</h3>"; echo $result = $a ** $b; ?>
2. PHP Increment / Decrement Operators
The PHP increment operators are used to increment a variable’s value.
The PHP decrement operators are used to decrement a variable’s value.
Operator | Name | Description |
---|---|---|
++$x | Pre-increment | Increments $x by one, then returns $x |
$x++ | Post-increment | Returns $x, then increments $x by one |
– -$x | Pre-decrement | Decrements $x by one, then returns $x |
$x- – | Post-decrement | Returns $x, then decrements $x by one |
3. PHP Assignment Operators
The PHP assignment operators are used with numeric values to write a value to a variable.
The basic assignment operator in PHP is “=”. It means that the left operand gets set to the value of the assignment expression on the right.
Assignment | Same as… | Description |
---|---|---|
x = y | x = y | The left operand gets set to the value of the expression on the right |
x += y | x = x + y | Addition |
x -= y | x = x – y | Subtraction |
x *= y | x = x * y | Multiplication |
x /= y | x = x / y | Division |
x %= y | x = x % y | Modulus |
Example:
<?php echo "a = ". $a = 20; echo "<br>b = ". $b = 5; echo "<h2>Assignment Oprators</h2>"; echo "<h3>Assign</h3>"; //echo $result = $a + $b; echo $a = $b; echo "<h3>Addition</h3>"; //echo $a = $a + $b; echo "a += b ==> " . $a += $b; echo "<h3>Subtraction</h3>"; //echo $a = $a - $b; echo "a -= b ==> " . $a -= $b; echo "<h3>Multiplication</h3>"; //echo $a = $a * $b; echo "a *= b ==> " . $a *= $b; echo "<h3>Division</h3>"; //echo $a = $a / $b; echo "a /= b ==> " . $a /= $b; echo "<h3>Modulus</h3>"; //echo $a = $a % $b; echo "a %= b ==> " . $a %= $b; ?>
4. PHP Comparison Operators
The PHP comparison operators are used to compare two values (number or string):
Operator | Name | Example | Result |
---|---|---|---|
== | Equal | $x == $y | Returns true if $x is equal to $y |
=== | Identical | $x === $y | Returns true if $x is equal to $y, and they are of the same type |
!= | Not equal | $x != $y | Returns true if $x is not equal to $y |
<> | Not equal | $x <> $y | Returns true if $x is not equal to $y |
!== | Not identical | $x !== $y | Returns true if $x is not equal to $y, or they are not of the same type |
> | Greater than | $x > $y | Returns true if $x is greater than $y |
< | Less than | $x < $y | Returns true if $x is less than $y |
>= | Greater than or equal to | $x >= $y | Returns true if $x is greater than or equal to $y |
<= | Less than or equal to | $x <= $y | Returns true if $x is less than or equal to $y |
<=> | Spaceship | $x <=> $y | Returns an integer less than, equal to, or greater than zero, depending on if $x is less than, equal to, or greater than $y. Introduced in PHP 7. |
5. PHP String Operators
PHP has two operators that are specially designed for strings.
Operator | Name | Example | Result |
---|---|---|---|
. | Concatenation | $txt1 . $txt2 | Concatenation of $txt1 and $txt2 |
.= | Concatenation assignment | $txt1 .= $txt2 | Appends $txt2 to $txt1 |
6. PHP Logical Operators
The PHP logical operators are used to combine conditional statements.
Operator | Name | Example | Result |
---|---|---|---|
and | And | $x and $y | True if both $x and $y are true |
or | Or | $x or $y | True if either $x or $y is true |
xor | Xor | $x xor $y | True if either $x or $y is true, but not both |
&& | And | $x && $y | True if both $x and $y are true |
|| | Or | $x || $y | True if either $x or $y is true |
! | Not | !$x | True if $x is not true |
Example:
<html> <head> <title>Logical Operators</title> </head> <body> <?php $a = 42; $b = 0; if( $a && $b ) { echo "TEST1 : Both a and b are true<br/>"; }else{ echo "TEST1 : Either a or b is false<br/>"; } if( $a and $b ) { echo "TEST2 : Both a and b are true<br/>"; }else{ echo "TEST2 : Either a or b is false<br/>"; } if( $a || $b ) { echo "TEST3 : Either a or b is true<br/>"; }else{ echo "TEST3 : Both a and b are false<br/>"; } if( $a or $b ) { echo "TEST4 : Either a or b is true<br/>"; }else { echo "TEST4 : Both a and b are false<br/>"; } $a = 10; $b = 20; if( $a ) { echo "TEST5 : a is true <br/>"; }else { echo "TEST5 : a is false<br/>"; } if( $b ) { echo "TEST6 : b is true <br/>"; }else { echo "TEST6 : b is false<br/>"; } if( !$a ) { echo "TEST7 : a is true <br/>"; }else { echo "TEST7 : a is false<br/>"; } if( !$b ) { echo "TEST8 : b is true <br/>"; }else { echo "TEST8 : b is false<br/>"; } ?> </body> </html>
PHP Array Operators
The PHP array operators are used to compare arrays.
Operator | Name | Example | Result |
---|---|---|---|
+ | Union | $x + $y | Union of $x and $y |
== | Equality | $x == $y | Returns true if $x and $y have the same key/value pairs |
=== | Identity | $x === $y | Returns true if $x and $y have the same key/value pairs in the same order and of the same types |
!= | Inequality | $x != $y | Returns true if $x is not equal to $y |
<> | Inequality | $x <> $y | Returns true if $x is not equal to $y |
!== | Non-identity | $x !== $y | Returns true if $x is not identical to $y |
PHP Conditional Assignment Operators
The PHP conditional assignment operators are used to set a value depending on conditions:
Operator | Name | Example | Result |
---|---|---|---|
?: | Ternary | $x = expr1 ? expr2 : expr3 | Returns the value of $x. The value of $x is expr2 if expr1 = TRUE. The value of $x is expr3 if expr1 = FALSE |
?? | Null coalescing | $x = expr1 ?? expr2 | Returns the value of $x. The value of $x is expr1 if expr1 exists, and is not NULL. If expr1 does not exist, or is NULL, the value of $x is expr2. Introduced in PHP 7 |
Conditional statement in PHP
PHP decision statements are used to make a decision, based on some conditions.
Type of Decision-Making Statements:
Here are different types of Decision Making Statements in PHP.
- if statement
- if…else statement
- if…elseif…else statement
- switch statement
if Statement:
This statement allows us to set a condition. On being TRUE, the following block of code enclosed within the if clause will be executed.
Syntax :
if (condition){ // if TRUE then execute this code }
Example:
<?php $x = 12; if ( $x > 0) { echo "The number is positive" ; } ?> |
Output:
The number is positive
Flowchart:
Ternary Operators
In addition to all this conditional statements, PHP provides a shorthand way of writing if…else, called Ternary Operators. The statement uses a question mark (?) and a colon (:) and takes three operands: a condition to check, a result for TRUE and a result for FALSE.
Syntax:
(condition) ? if TRUE execute this : otherwise execute this;
Example:
<?php $x = -12; if ( $x > 0) { echo "The number is positive \n" ; } else { echo "The number is negative \n" ; } // This whole lot can be written in a // single line using ternary operator echo ( $x > 0) ? 'The number is positive' : 'The number is negative' ; ?> |
Output:
The number is negative The number is negative
The if….else statement
if…elseif…else Statement
This allows us to use multiple if…else statements. We use this when there are multiple conditions of TRUE cases.
Syntax:
if (condition) { // if TRUE then execute this code } elseif { // if TRUE then execute this code } elseif { // if TRUE then execute this code } else { // if FALSE then execute this code }
Example:
<?php $x = "August" ; if ( $x == "January" ) { echo "Happy Republic Day" ; } elseif ( $x == "August" ) { echo "Happy Independence Day!!!" ; } else { echo "Nothing to show" ; } ?> |
Output:
Happy Independence Day!!!
Flowchart:
The switch…case statement
The “switch” performs in various cases i.e., it has various cases to which it matches the condition and appropriately executes a particular case block. It first evaluates an expression and then compares with the values of each case. If a case matches then the same case is executed. To use switch, we need to get familiar with two different keywords namely, break and default.
- The break statement is used to stop the automatic control flow into the next cases and exit from the switch case.
- The default statement contains the code that would execute if none of the cases match.
Syntax:
switch(n) { case statement1: code to be executed if n==statement1; break; case statement2: code to be executed if n==statement2; break; case statement3: code to be executed if n==statement3; break; case statement4: code to be executed if n==statement4; break; ...... default: code to be executed if n != any case;
Example:
<?php $n = "February" ; switch ( $n ) { case "January" : echo "Its January" ; break ; case "February" : echo "Its February" ; break ; case "March" : echo "Its March" ; break ; case "April" : echo "Its April" ; break ; case "May" : echo "Its May" ; break ; case "June" : echo "Its June" ; break ; case "July" : echo "Its July" ; break ; case "August" : echo "Its August" ; break ; case "September" : echo "Its September" ; break ; case "October" : echo "Its October" ; break ; case "November" : echo "Its November" ; break ; case "December" : echo "Its December" ; break ; default : echo "Doesn't exist" ; } ?> |
Output:
Its February
Flowchart:
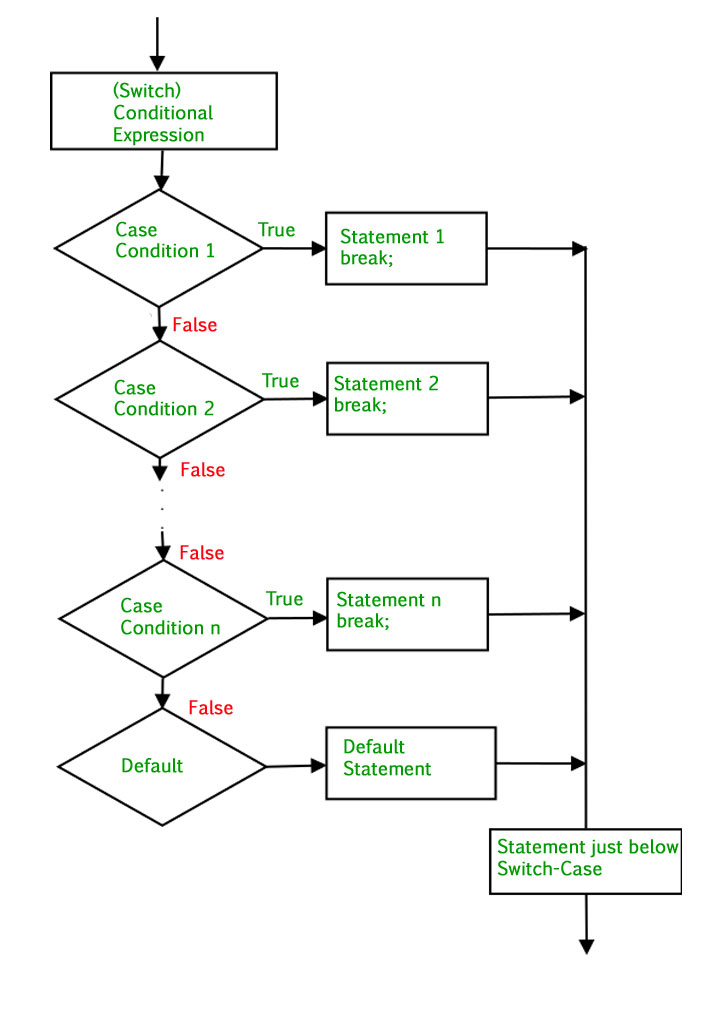
PHP switch Statement
The switch-case statement is an alternative to the if-elseif-else statement, which does almost the same thing. In many occasions, you may want to compare the same variable (or expression) with many different values, and execute a different piece of code depending on which value it equals to. This is exactly what the switch
statement is for.
The switch
statement is used to perform different actions based on different conditions.
Use the switch
statement to select one of many blocks of code to be executed.
Syntax
switch (n) {
case label1:
code to be executed if n=label1;
break;
case label2:
code to be executed if n=label2;
break;
case label3:
code to be executed if n=label3;
break;
…
default:
code to be executed if n is different from all labels;
}
This is how it works: First we have a single expression n (most often a variable), that is evaluated once. The value of the expression is then compared with the values for each case in the structure. If there is a match, the block of code associated with that case is executed. Use break
to prevent the code from running into the next case automatically. The default
statement is used if no match is found.
Example
<?php $favcolor = "red";switch ($favcolor) { case "red": echo "Your favorite color is red!"; break; case "blue": echo "Your favorite color is blue!"; break; case "green": echo "Your favorite color is green!"; break; default: echo "Your favorite color is neither red, blue, nor green!"; } ?>
The following two examples are two different ways to write the same thing, one using a series of if
and elseif
statements, and the other using the switch
statement:
Example #1 switch
structure
<?php
if ($i == 0) {
echo "i equals 0";
} elseif ($i == 1) {
echo "i equals 1";
} elseif ($i == 2) {
echo "i equals 2";
}
switch ($i) { case 0: echo "i equals 0"; break; case 1: echo "i equals 1"; break; case 2: echo "i equals 2"; break; } ?>
Important points to be noticed about switch case:
- The default is an optional statement. Even it is not important, that default must always be the last statement.
- There can be only one default in a switch statement. More than one default may lead to a Fatal error.
- Each case can have a break statement, which is used to terminate the sequence of statement.
- The break statement is optional to use in switch. If break is not used, all the statements will execute after finding matched case value.
- PHP allows you to use number, character, string, as well as functions in switch expression.
- Nesting of switch statements is allowed, but it makes the program more complex and less readable.
- You can use semicolon (;) instead of colon (:). It will not generate any error.
PHP Loops
Often when you write code, you want the same block of code to run over and over again a certain number of times. So, instead of adding several almost equal code-lines in a script, we can use loops.
PHP Loops are used to execute the same block of code again and again until a certain condition is met.
Types of Loops
In PHP, we have the following loop types:
while
– loops through a block of code as long as the specified condition is truedo...while
– loops through a block of code once, and then repeats the loop as long as the specified condition is truefor
– loops through a block of code a specified number of timesforeach
– loops through a block of code for each element in an array
for Loop
The for loop repeats a block of code until a certain condition is met. It is typically used to execute a block of code for certain number of times.
Syntax
for(initialization; condition; increment){
// Code to be executed
}
while Loop
The while loop executes a block of code as long as the specified condition is true.
Syntax
while (condition is true) {
code to be executed;
}
The number is: 2
The number is: 3
The number is: 4
The number is: 5
do…while Loop
The do…while statement will execute a block of code at least once – it then will repeat the loop as long as a condition is true.
Syntax
do {
code to be executed;
}
while (condition);
The number is: 6
PHP Array
What is an Array?
An array is a special variable, which can hold or stores multiple values in one single variable:
If you have a list of items (a list of car names, for example), storing the cars in single variables could look like this:
$cars1 = “Volvo”;
$cars2 = “BMW”;
$cars3 = “Toyota”;
However, what if you want to loop through the cars and find a specific one? And what if you had not 3 cars, but 300?
The solution is to create an array!
An array can hold many values under a single name, and you can access the values by referring to an index number.
Create an Array in PHP
In PHP, the array()
function is used to create an array:
array();
Example
<?php
$cars = array("Volvo", "BMW", "Toyota");
?>
3 ways to create Array:-
Example:-
<?php // PHP arrays - stores multiple values in single variable // first method $arr1 = array('red', 'yellow','blue','pink'); echo "<pre>"; print_r($arr1); echo "</pre>"; // second method $arr2 = ['red', 'yellow','blue','pink']; echo "<pre>"; print_r($arr2); echo "</pre>"; // third method $arr3[0] = 'red'; $arr3[1] = 'yellow'; $arr3[2] = 'blue'; $arr3[3] = 'pink'; $arr3[] = 'orange'; echo "<pre>"; print_r($arr3); echo "</pre>"; ?>
In above example, all three methods, have been explained.
Types of Array
In PHP, there are three types of arrays:
-
- Indexed or Numeric arrays
– Arrays with a numeric index
-
- Associative arrays
– Arrays with named keys
-
- Multidimensional arrays
– Arrays containing one or more arrays
1. PHP Indexed or Numeric Arrays
These type of arrays can be used to store any type of elements, but an index is always a number. By default, the index starts at zero.
There are three ways to create indexed arrays:
The index can be assigned automatically (index always starts at 0), like this:
$cars = array("Volvo", "BMW", "Toyota");
or the index can be assigned manually:
$cars = [“Volvo”, “BMW”, “Toyota”];
or the index can be assigned manually:
$cars[0] = "Volvo";
$cars[1] = "BMW";
$cars[2] = "Toyota";
The following example creates an indexed array named $cars, assigns three elements to it, and then prints a text containing the array values:
Example
<?php
$cars = array("Volvo", "BMW", "Toyota");
echo "I like " . $cars[0] . ", " . $cars[1] . " and " . $cars[2] . ".";
?>
Get The Length of an Array – The count() Function
The count()
function is used to return the length (the number of elements) of an array:
Example
<?php
$cars = array("Volvo", "BMW", "Toyota");
echo count($cars);
?>
Loop Through an Indexed Array/ Traversing
We can traverse an indexed array using loops in PHP. We can loop through the indexed array in two ways.
-
- By using for loop and
- By using foreach.
1. Using for loop
To loop through and print all the values of an indexed array, you could use a for
loop, like this:
<?php $cars = array("Volvo", "BMW", "Toyota"); $arrlength = count($cars); for($x = 0; $x < $arrlength; $x++) { echo $cars[$x]; echo "<br>"; } ?>
2. Using foreach loop
<?php
$cars = array("Volvo", "BMW", "Toyota"); foreach($cars as $value) { echo $value; echo "<br>"; } ?>
3. Multidimensional Arrays
We have so far described arrays that are a single list of key/value pairs. However, sometimes you want to store values with more than one key. For this, we have multidimensional arrays.
PHP – Multidimensional Arrays
A multidimensional array is an array containing one or more arrays.
PHP supports multidimensional arrays that are two, three, four, five, or more levels deep. However, arrays more than three levels deep are hard to manage for most people.
The dimension of an array indicates the number of indices you need to select an element.
- For a two-dimensional array you need two indices to select an element
- For a three-dimensional array you need three indices to select an element
PHP – Two-dimensional Arrays
A two-dimensional array is an array of arrays (a three-dimensional array is an array of arrays of arrays).
First, take a look at the following table:
Name | Stock | Sold |
---|---|---|
Volvo | 22 | 18 |
BMW | 15 | 13 |
Saab | 5 | 2 |
Land Rover | 17 | 15 |
We can store the data from the table above in a two-dimensional array, like this:
$cars = array (
array("Volvo",22,18),
array("BMW",15,13),
array("Saab",5,2),
array("Land Rover",17,15)
);
Now the two-dimensional $cars array contains four arrays, and it has two indices: row and column.
To get access to the elements of the $cars array we must point to the two indices (row and column):
Example
<?php
echo $cars[0][0].": In stock: ".$cars[0][1].", sold: ".$cars[0][2].".<br>";
echo $cars[1][0].": In stock: ".$cars[1][1].", sold: ".$cars[1][2].".<br>";
echo $cars[2][0].": In stock: ".$cars[2][1].", sold: ".$cars[2][2].".<br>";
echo $cars[3][0].": In stock: ".$cars[3][1].", sold: ".$cars[3][2].".<br>";
?>
We can also put a for
loop inside another for
loop to get the elements of the $cars array (we still have to point to the two indices):
Example
<?php
for ($row = 0; $row < 4; $row++) {
echo "<p><b>Row number $row</b></p>";
echo "<ul>";
for ($col = 0; $col < 3; $col++) {
echo "<li>".$cars[$row][$col]."</li>";
}
echo "</ul>";
}
?>
|
Output:
Dave Punk email-id is: davepunk@gmail.com John Flinch mobile number is: 9875147536
Traversing Multidimensional Arrays: We can traverse through the multidimensional array using for and foreach loop in a nested way. That is, one for loop for the outer array and one for loop for the inner array.
|
Output:
Dave Punk mob : 2584369721 email : montysmith@gmail.com John Flinch mob : 9875147536 email : johnflinch@gmail.com
Magic Constants
It starts with a double underscore
- __LINE__
- __FILE__
- __DIR__
- __FUNCTION__
- __CLASS__
- __METHOD__
1. __LINE__
This gives the current line number.
Code:
<?php
//example to demonstrate PHP magic constant __LINE__
echo 'I am at Line number '. __LINE__;
?>
Output:
2.__FILE__
This gives the filename along with the file path of the file. It can be used to include a file in a script.
Code:
<?php
//example to demonstrate PHP magic constant __FILE__
echo 'FILE NAME '. __FILE__;
?>
Output:
3.__DIR__
This gives the directory of the file.
Code:
<?php
//example to demonstrate PHP magic constant __DIR__
echo 'DIRNAME '. __DIR__;
?>
Output:
directory name: E:\xampp\php
4. __FUNCTION__
This gives the name of the function in which it is declared. It is case sensitive.
Code:
<?php
// example to demonstrate the magic constant __FUNCTION__
function show() {
echo 'In the function '.__FUNCTION__;
}
show();
?>
Output:
5. __METHOD__ , __CLASS__
This gives the name of the method and the name of the class in which it is declared. In the below example, we have defined the MainClass and two methods within it, the show method and the test method. Inside the show method, we have printed the __CLASS__, which gives the class name and inside the test method, we have printed the __METHOD__, which gives the method name, test.
Code:
<?php
// example to demonstrate the magic constant __CLASS__ and __METHOD__
class MainClass
{
function show() {
echo "<br>".__CLASS__;
}
function test() {
echo "<br>".__METHOD__;
}
}
$obj = new MainClass;
echo $obj->show();
echo $obj->test();
?>
Output: